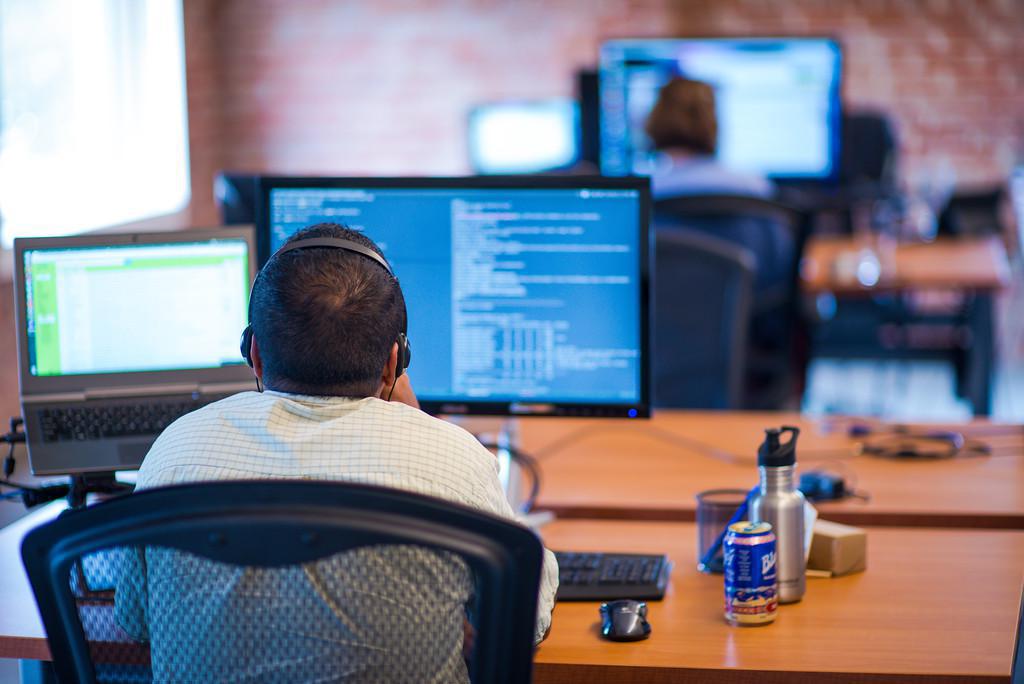
Note: In between the time I originally wrote this post and it getting published, a ticket and pull request were opened in Django to remove allow_unsaved_instance_assignment and move validation to the model save() method, which makes much more sense anyways. It's likely this will even be backported to Django 1.8.4. So, if you're using a version of Django that doesn't require this, hopefully you'll never stumble across this post in the first place! If this is still an issue for you, here's the original post:
In versions of Django prior to 1.8, it was easy to construct "sample" model data by putting together a collection of related model objects, even if none of those objects was saved to the database. Django 1.8 - 1.8.3 adds a restriction that prevents this behavior. Errors such as this are generally a sign that you're encountering this issue:
ValueError: Cannot assign "...": "MyRelatedModel" instance isn't saved in the database.
The justification for this is that, previously, unsaved foreign keys were silently lost if they were not saved to the database. Django 1.8 does provide a backwards compatibility flag to allow working around the issue. The workaround, per the Django documentation, is to create a new ForeignKey field that removes this restriction, like so:
class UnsavedForeignKey(models.ForeignKey):
# A ForeignKey which can point to an unsaved object
allow_unsaved_instance_assignment = True
class Book(models.Model):
author = UnsavedForeignKey(Author)
This may be undesirable, however, because this approach means you lose all protection for all uses of this foreign key, even if you want Django to ensure foreign key values have been saved before being assigned in some cases.
There is a middle ground, not immediately obvious, that involves changing this attribute temporarily during the assignment of an unsaved value and then immediately changing it back. This can be accomplished by writing a context manager to change the attribute, for example:
import contextlib
@contextlib.contextmanager
def allow_unsaved(model, field):
model_field = model._meta.get_field(field)
saved = model_field.allow_unsaved_instance_assignment
model_field.allow_unsaved_instance_assignment = True
yield
model_field.allow_unsaved_instance_assignment = saved
To use this decorator, surround any assignment of an unsaved foreign key value with the context manager as follows:
with allow_unsaved(MyModel, 'my_fk_field'):
my_obj.my_fk_field = unsaved_instance
The specifics of how you access the field to pass into the context manager are important; any other way will likely generate the following error:
RelatedObjectDoesNotExist: MyModel has no instance.
While strictly speaking this approach is not thread safe, it should work for any process-based worker model (such as the default "sync" worker in Gunicorn).
This took a few iterations to figure out, so hopefully it will (still) prove useful to someone else!