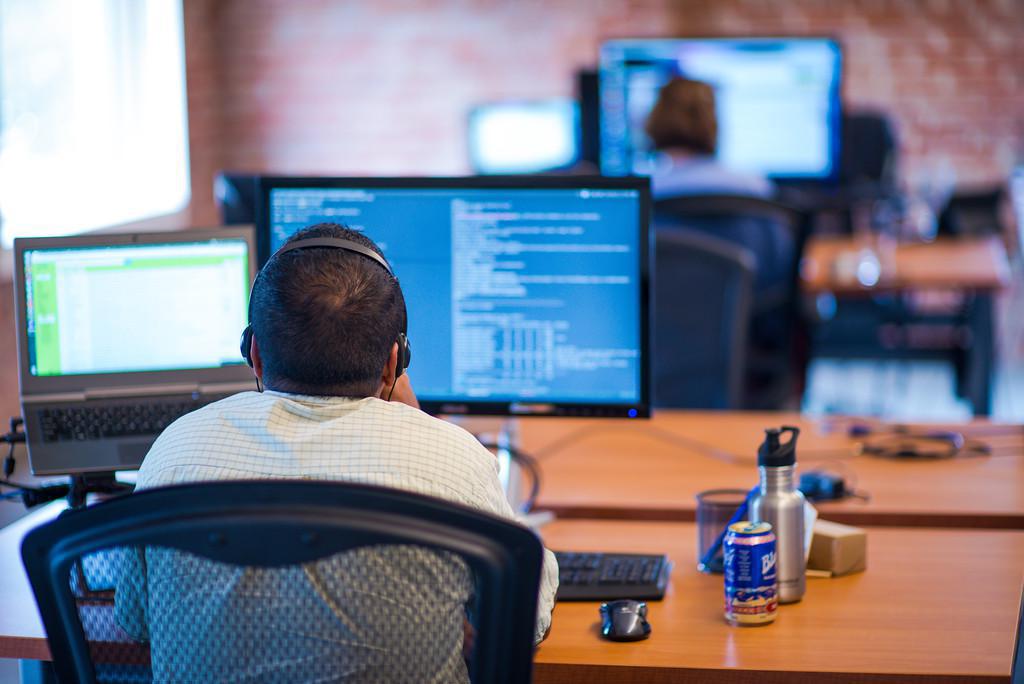
One of the things I like best about Django is how easy its ORM makes it to work with databases. Too bad Django is only for web applications. Sure, you could deploy a Django app and then make use of it from a non-web application using a REST API, but that would be too awkward.
But there is an easy way to use Django without the web! Here's the trick - write your application as Django management commands. Then you can run it from the command line. Just like 'manage.py syncdb' or 'manage.py migrate', you can run 'manage.py my_own_application' and your application has access to the full power of Django ORM.
Adding a new Django management command is surprisingly easy:
- Add a management/commands directory to your application.
- Create a anything.py file containing a class that extends django.core.management.base.BaseCommand or a subclass.
- Write a handle method that runs your application
- Run 'manage.py anything'
Here's an example of a trivial command:
from django.core.management.base import BaseCommand
class Command(BaseCommand):
def handle(self, *args, **kwargs):
print "Hello, world"
Create a management/commands directory in your application and save this there as 'hello.py'.
Now try it:
$ ./manage.py hello
Hello, world
$
How about doing something useful? Here's an example that prints out all of your invoices, so you can see how easy it is to access your data:
from django.core.management.base import BaseCommand
from appname.models import Invoice
class Command(BaseCommand):
def handle(self, *args, **kwargs):
print "Invoices"
for invoice in Invoice.objects.order_by('date'):
print u"%s %s" % (invoice.date, invoice.summary)
I've used custom management commands to do things like importing data where something more complicated than loading a fixture was needed.
For more details, see the Django documentation.